Node.js를 사용하여 SFTP에 연결하고, 파일을 리스트업하며, 업로드 및 다운로드하는 방법을 배웁니다.
이 기사를 다 읽고 나면, 마침내 여러분은 Node.js 코드작성에 익숙해져 SFTP 서버에 접속해 작업이 가능하게 되어, 우리의 도움은 더이상 필요없게 될 것입니다. SFTP는 널리 사용되는 표준 프로토콜이며 파일 및 데이터의 안전한 전송에 중점을 둔 보안 프로토콜이지만 서버와의 연결을 설정할 때 몇 가지 단계를 완료해야합니다. 자, 그럼 함께 해봅시다!
필요사항
첫단계: ssh2-sftp-client
는 node.js용 SFTP 클라이언트로, SSH2의 래퍼(wrapper)로서 SFTP 관련 기능을 고도로 추상화한 것입니다. 설치할 준비가 되면 먼저 다음 코드를 수동으로 실행합니다.
npm install ssh2-sftp-client@^8.0.0
또는 package.json파일을 추가한 다음 종속성을 선언합니다
{
"dependencies": {
"ssh2-sftp-client": "^8.0.0"
}
}
그리고, 아래를 실행합니다 :
npm install
SFTP에 연결
이 기사에서는 예로서 SFTPTOGO_URL이라는 환경 변수를 사용합니다. 이 환경 변수에는 SFTP 서버에 연결하는 데 필요한 모든 정보가 다음과 같은 URI 형식으로 포함됩니다: sftp://user:password@host. 이 변수는 URI.parse를 사용합니다여 URI 부분을 추출하고 리모트 서버의 호스트 키는 디폴트로 ~/.ssh/known_hosts
를 사용하여 검증합니다.
let Client = require('ssh2-sftp-client');
class SFTPClient {
constructor() {
this.client = new Client();
}
async connect(options) {
console.log(`Connecting to ${options.host}:${options.port}`);
try {
await this.client.connect(options);
} catch (err) {
console.log('Failed to connect:', err);
}
}
async disconnect() {
await this.client.end();
}
}
파일 리스트 표시
연결이 설정되면 리모트 SFTP 서버의 파일들을 나열해서 나타낼 수 있습니다. 이것은, ssh2-sftp-client의 list 메소드를 호출해 돌려주어지는 배열을 반복 처리하는 것으로, path 인수로 발견된 파일에 대응할 수가 있습니다. 이 예에서는 래퍼 함수가 리모트 경로에서 발견된 파일을 단순히 출력합니다.
async listFiles(remoteDir, fileGlob) {
console.log(`Listing ${remoteDir} ...`);
let fileObjects;
try {
fileObjects = await this.client.list(remoteDir, fileGlob);
} catch (err) {
console.log('Listing failed:', err);
}
const fileNames = [];
for (const file of fileObjects) {
if (file.type === 'd') {
console.log(`${new Date(file.modifyTime).toISOString()} PRE ${file.name}`);
} else {
console.log(`${new Date(file.modifyTime).toISOString()} ${file.size} ${file.name}`);
}
fileNames.push(file.name);
}
return fileNames;
}
파일 업로드
다음 단계는 파일 업로드입니다. 클라이언트 객체의 put
함수를 사용해, 로컬 파일의 패스와 리모트 패스를 건네줍니다 (업로드 후에 파일은 리모트 패스에 놓입니다). 함수 호출은 다음과 같습니다: await client.uploadFile("./local.txt", "./remote.txt");
async uploadFile(localFile, remoteFile) {
console.log(`Uploading ${localFile} to ${remoteFile} ...`);
try {
await this.client.put(localFile, remoteFile);
} catch (err) {
console.error('Uploading failed:', err);
}
}
파일 다운로드
마지막 단계로 파일을 다운로드합니다. 클라이언트 객체의 get
함수를 사용하여 리모트 파일의 경로와 다운로드한 파일을 저장하는 로컬 경로를 전달합니다. 이것은 다음과 같습니다: await client.downloadFile("./remote.txt", "./download.txt")
async downloadFile(remoteFile, localFile) {
console.log(`Downloading ${remoteFile} to ${localFile} ...`);
try {
await this.client.get(remoteFile, localFile);
} catch (err) {
console.error('Downloading failed:', err);
}
}
마무리
위의 단계를 다 진행하면 모든 프로세스가 완료됩니다. 마지막으로 프로그램을 처음부터 끝까지 실행하고 싶다면 다음 코드를 복사하여 main.js
로 저장하십시오:
// sftp.js
//
// Use this sample code to connect to your SFTP To Go server and run some file operations using Node.js.
//
// 1) Paste this code into a new file (sftp.js)
//
// 2) Install dependencies
// npm install ssh2-sftp-client@^8.0.0
//
// 3) Run the script
// node sftp.js
//
// Compatible with Node.js >= v12
// Using ssh2-sftp-client v8.0.0
let Client = require('ssh2-sftp-client');
class SFTPClient {
constructor() {
this.client = new Client();
}
async connect(options) {
console.log(`Connecting to ${options.host}:${options.port}`);
try {
await this.client.connect(options);
} catch (err) {
console.log('Failed to connect:', err);
}
}
async disconnect() {
await this.client.end();
}
async listFiles(remoteDir, fileGlob) {
console.log(`Listing ${remoteDir} ...`);
let fileObjects;
try {
fileObjects = await this.client.list(remoteDir, fileGlob);
} catch (err) {
console.log('Listing failed:', err);
}
const fileNames = [];
for (const file of fileObjects) {
if (file.type === 'd') {
console.log(`${new Date(file.modifyTime).toISOString()} PRE ${file.name}`);
} else {
console.log(`${new Date(file.modifyTime).toISOString()} ${file.size} ${file.name}`);
}
fileNames.push(file.name);
}
return fileNames;
}
async uploadFile(localFile, remoteFile) {
console.log(`Uploading ${localFile} to ${remoteFile} ...`);
try {
await this.client.put(localFile, remoteFile);
} catch (err) {
console.error('Uploading failed:', err);
}
}
async downloadFile(remoteFile, localFile) {
console.log(`Downloading ${remoteFile} to ${localFile} ...`);
try {
await this.client.get(remoteFile, localFile);
} catch (err) {
console.error('Downloading failed:', err);
}
}
async deleteFile(remoteFile) {
console.log(`Deleting ${remoteFile}`);
try {
await this.client.delete(remoteFile);
} catch (err) {
console.error('Deleting failed:', err);
}
}
}
(async () => {
const parsedURL = new URL(process.env.SFTPTOGO_URL);
const port = parsedURL.port || 22;
const { host, username, password } = parsedURL;
//* Open the connection
const client = new SFTPClient();
await client.connect({ host, port, username, password });
//* List working directory files
await client.listFiles(".");
//* Upload local file to remote file
await client.uploadFile("./local.txt", "./remote.txt");
//* Download remote file to local file
await client.downloadFile("./remote.txt", "./download.txt");
//* Delete remote file
await client.deleteFile("./remote.txt");
//* Close the connection
await client.disconnect();
})();
마지막으로, 아래 명령을 실행하시면 됩니다:
node main.js
축하드립니다! Node.js를 이용하여 SFTP에 연결되었습니다!
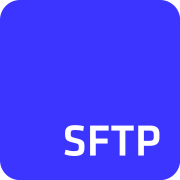
또한, Github에 있는 저희 리포지토리에서 더 많은 코드 샘플들을 체크해 보세요.