Today, the ability to securely transfer and manage files is not just a convenience but a necessity, especially for professionals dealing with sensitive data or managing complex IT environments.
This guide will help you effectively connect and use SFTP with PowerShell to handle data.
Here's a snapshot of what's in store:
- Environment setup: Prepare your system with the tools and configurations to use SFTP with PowerShell.
- Establishing connections: How to securely connect to your SFTP server using SFTP To Go.
- Practical file management: Dive into real-world applications, learning to manage file uploads, downloads, and synchronization.
- Best practices and security: Tips and practices to maintain efficient, secure, and reliable file transfer processes.
What makes SFTP and PowerShell click
In case you’re second guessing yourself, let's first understand why SFTP and PowerShell go together like beer and ice-cold.
What’s great about SFTP?
- Encryption: SFTP encrypts both the data and commands, providing security against unauthorized access during the transfer.
- Versatility: It allows a wide range of operations like file uploads, downloads, and directory listings.
- Reliability: SFTP ensures that the data is accurately and completely transferred.
And PowerShell?
- Automation: PowerShell can automate repetitive tasks, saving time and reducing errors.
- Flexibility: It can interact with various services and protocols, including SFTP.
- Advanced scripting: PowerShell can handle complex workflows and processes with its advanced scripting capabilities.
Why combine SFTP and PowerShell?
When you use SFTP within PowerShell, you're combining the security of SFTP with the automation and scripting power of PowerShell. This combination is beneficial for tasks like:
- Automating file transfers: You can automate securely transferring files to and from servers.
- Scheduled backups: Set up scripts to regularly back up important files to an SFTP server.
- Data synchronization: Keep data synchronized between different locations in a secure and automated way.
Step 1: Preparing your environment
It's time to set up your environment for using SFTP with PowerShell. This step lays the groundwork for all the cool stuff we will do later. Let's get your system ready!
1.1 Checking your PowerShell version
First, let's ensure you have PowerShell on your machine. Here's how you can check your PowerShell version:
- Open PowerShell by searching for it in your Windows search bar.
- Type Get-Host and press Enter.
- Look for the version number in the output.
If you're running Windows 10 or later, you likely have PowerShell pre-installed. No worries if you're on an older version or your PowerShell needs updating. You can download the PowerShell from the GitHub Repository.
1.2 Installing Posh-SSH
Now, onto the fun part, which is installing Posh-SSH. This PowerShell module adds SFTP functionality to PowerShell. Here's a step-by-step guide to install it:
- Open PowerShell as an Administrator: Right-click on PowerShell and select Run as administrator.
- Install the module: Enter the command:
Install-Module -Name Posh-SSH
Hit Enter. If prompted to trust the repository, type Y and press Enter again.
- Verify the installation: To make sure Posh-SSH is installed, you can list all installed modules with the following command:
Get-Module -ListAvailable Posh-SSH
1.3 Setting execution policy
PowerShell has something called an execution policy to control the running of scripts. You might need to adjust this to use Posh-SSH. Here's how:
- Check current policy: Type Get-ExecutionPolicy and hit Enter.
- Change the policy: If the policy is restrictive, change it by typing:
Set-ExecutionPolicy RemoteSigned
This allows you to run scripts written on your local computer and signed scripts from the internet.
- Confirm the change: Type Y and hit Enter.
Step 2: Establishing connection to SFTP To Go
Great job getting your environment ready! Now, let's connect to your SFTP To Go server using PowerShell. Two common methods to establish this connection are username and password or SSH key authentication.
2.1 Username and password authentication
If you opt for the username and password method, it's straightforward and great for getting started quickly. Here's how you do it:
- Open PowerShell: You know the drill! Fire up your PowerShell.
- Start a new SFTP session: Enter the following command:
$Credential = Get-Credential
New-SFTPSession -ComputerName "SFTPToGoServerAddress" -Credential $Credential
Replace SFTPToGoServerAddress with your actual SFTP To Go server address.
3. Enter your credentials: A prompt will appear for your username and password. Enter your SFTP To Go credentials here.
- Username: Enter your SFTP To Go username.
- Password: Enter your SFTP To Go password.
4. Confirm the connection: Once connected, you can list the existing sessions to confirm by running:
Get-SFTPSession
2.2 SSH key authentication
SSH key authentication is the way to a more secure connection, especially in production environments. Here's how you set it up:
- Ensure you have an SSH key: If you don't already have an SSH key, you'll need to create one. You can follow these instructions for generating SSH keys on Windows.
- Open PowerShell and start the session: Run the following command:
New-SFTPSession -ComputerName " SFTPToGoServerAddress" -Credential (New-Object System.Management.Automation.PSCredential ("Username", (New-Object System.Security.SecureString))) -KeyFile "C:\key"
Replace SFTPToGoServerAddress, Username, and C:\key with your actual server address, username, and path to your SSH key.
3. Verify the connection: Check your active SFTP sessions with:
Get-SFTPSession
2.3 Troubleshooting Tips
- Connection issues: Double-check your credentials and server address if you encounter any issues while trying to connect. Ensure there's no firewall or network issue blocking the connection.
- Permission denied: If you see this error during SSH key authentication, ensure your private key is in the correct format and the path is correctly specified.
Step 3: File management made easy
Now that you're connected to your SFTP To Go server with PowerShell, it's time to dive into SFTP's core, managing files. PowerShell makes these tasks straightforward and efficient, whether you're uploading, downloading, or organizing your files. Let's explore how you can perform various file operations with ease.
3.1 Uploading files to SFTP To Go
Uploading files to your SFTP server is a common task. Here's how to do it in PowerShell:
- Locate the file you want to upload: Find the file's path on your local machine. For example, C:\test.txt.
- Choose your destination on the server: Decide where the file will go on your SFTP server. It could be something like /uploads/.
- Execute the upload command: Use the following command to upload the file:
$SessionId = (Get-SFTPSession).SessionId
Set-SFTPFile -SessionId $SessionId -LocalFile "C:\test.txt" -RemotePath "/uploads/test.txt"
Replace the paths with your specific file and destination.
4. Check the upload: Optionally, you can verify that your file has been uploaded by listing the contents of the destination directory on the server.
3.2 Downloading files from SFTP To Go
Downloading files is just as straightforward. Here's how to do it:
- Find the file on the server: Identify the file you want to download and its path on the SFTP server.
- Choose a local destination: Decide where to save the file on your local machine.
- Use the download command: Execute the following command:
Get-SFTPFile -SessionId $SessionId -RemoteFile "/uploads/test.txt" -LocalPath "C:\test.txt"
Adjust the paths to match your file locations.
4. Verify the download: Check your local destination folder to ensure the file has been downloaded.
3.3 Listing and managing files on the server
Seeing what's on your SFTP server and managing these files can be done quickly:
- List files in a directory: To view files in a specific directory, use:
Get-SFTPChildItem -SessionId $SessionId -Path "/uploads/"
2. Creating directories: To create a new directory on the server, use:
New-SFTPItem -SessionId $SessionId -Path "/uploads/test" -ItemType Directory
3. Deleting files: To delete a file, use the following command:
Remove-SFTPItem -SessionId $SessionId -Path "/uploads/test.txt"
3.4 Handling large files and directories
Dealing with large files or numerous files can be a bit trickier, but PowerShell can handle it:
- Transferring large files: Break large files into smaller chunks or use compression before transferring.
- Bulk operations: For transferring multiple files, consider scripting the operations in a loop or using batch commands.
3.5 File management smart habits
- Regular backups: Use PowerShell scripts to automate regular backups of essential files to your SFTP server.
- Logging: Implement logging in your scripts to keep track of file operations, especially useful for troubleshooting and audit trails.
- Error handling: Incorporate error handling in your scripts to manage exceptions and unexpected scenarios gracefully.
Step 4: Best practices and pro tips
Having explored the various aspects of file management and advanced operations with SFTP in PowerShell, let's solidify your knowledge with some best practices and professional tips. These guidelines will help ensure your file transfers are not only efficient but also secure and reliable.
4.1 Secure credential management
Handling credentials securely is paramount in any scripting or automation task:
- Avoid hardcoding credentials: Never hardcode usernames and passwords in your scripts. Instead, use secure methods like environment variables or encrypted files.
- Windows credential manager: Consider storing your credentials in the Windows Credential Manager and accessing them in your PowerShell scripts for added security.
4.2 Efficient scripting
Write efficient and clean code for better performance and maintainability:
- Modular approach: Break your scripts into modules or functions, each performing a specific task. This makes your code more organized and reusable.
- Comment your code: Use comments to explain complex parts of your script. This is especially helpful when you return to the code after a long time or when sharing with others.
- Optimize performance: If you're dealing with many files, optimize your scripts to handle bulk operations efficiently.
4.3 Regular updates and patching
Keep your environment and tools up to date:
- Update PowerShell and modules: Regularly check for and install updates for PowerShell and modules like Posh-SSH to ensure you have the latest features and security patches.
- Stay informed: Stay updated with the latest PowerShell scripting and SFTP technologies to take advantage of new tools and practices.
Conclusion: integrating SFTP into your PowerShell workflow
You've embarked on a valuable journey into SFTP and PowerShell, unlocking essential skills that’ll do you proud.
Whether managing files for personal projects or handling sensitive data professionally, the knowledge you've gained here will undoubtedly enhance your efficiency and data security.
Are you looking to connect Connect To SFTP In C# instead? Go Here.
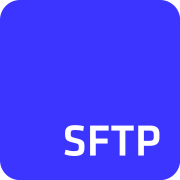
Frequently Asked Questions
Can I use PowerShell for automated SFTP file transfers in a business environment?
Absolutely! PowerShell, combined with SFTP, is an excellent choice for automating secure file transfers in a business environment. It's efficient for handling tasks like automated backups, data synchronization, and batch file transfers, ensuring both security and automation efficiency.
How secure is file transfer using SFTP and PowerShell?
SFTP is a secure method as it encrypts the data during transmission, preventing unauthorized access. When used with PowerShell, as long as you follow the best script security and credential management practices, your file transfers will be secure.
Is there a way to handle errors or interruptions during file transfers in PowerShell?
Yes, PowerShell scripts can be written to include error-handling mechanisms. You can use try-catch blocks to capture errors and implement logic to retry the transfer in case of interruptions, ensuring robust and reliable file operations.
Can I manage large files with PowerShell and SFTP?
PowerShell can handle large file transfers via SFTP. However, it's advisable to implement strategies like file compression or chunking large files into smaller parts for more efficient transfers, especially over slower network connections.
How can I ensure my PowerShell SFTP scripts are performant and not consuming excessive resources?
Optimize your scripts by processing files in batches, avoiding unnecessary loops, and using efficient PowerShell cmdlets to ensure your scripts are performant. Also, monitor the resource usage of your scripts during execution, especially in a production environment.
Are there any limitations to using PowerShell with SFTP?
The primary limitations would be related to the network bandwidth and the performance of the server hosting the SFTP service. PowerShell is quite capable and can handle complex scripting requirements for SFTP operations.
How can I schedule my PowerShell scripts to run at specific times?
You can run your PowerShell scripts at specified times using Windows Task Scheduler. This is particularly useful for regular tasks like backups or data synchronization.
Should I use a GUI-based SFTP client or PowerShell scripting?
It depends on your needs and skills. A GUI-based SFTP client is user-friendly and suitable for ad-hoc file transfers. In contrast, PowerShell scripting is ideal for automating repetitive tasks, handling bulk operations, and integrating file transfers into larger workflows.