Secure data transmission is essential in 2024. Lucky for us, SFTP (Secure File Transfer Protocol) is a reliable method for transferring files securely over the internet. It’s a trusted solution for businesses that value data security, especially when dealing with sensitive information.
SFTP To Go is a fully managed, efficient, and secure way to interact with SFTP servers, extending the capabilities of traditional SFTP by ensuring that file transfers are encrypted, fast.
If you're a .NET developer, integrating SFTP functionalities into your applications can be a critical requirement. We’re going to show you how to do this with SFTP To Go.
Guide overview
In this guide, you’ll explore the practical implementation of SFTP in C# using the SSH.NET library, a popular framework for handling network operations in .NET applications. You’ll also deal with two key components designed for SFTP operations with SFTP To Go in C#.
The first is the SFTPClient, a console application that allows users to interactively perform file operations like uploading, downloading, and managing files on our SFTP server. By the end of this guide, you’ll know how to set it up and perform these operations.
The second is the SFTPService, a class library project that provides the underlying functionalities necessary for SFTP interactions, including connecting to the server and handling file operations.
This split ensures a clear separation between the user interface, offered by the SFTPClient for direct interaction, and the core SFTP functionalities, encapsulated within the SFTPService, thereby promoting modularity and reusability in different C# projects.
So:
- SFTPClient: A console application for various file operations using SFTP To Go.
- SFTPService: A class library project containing functionalities for connecting, uploading, downloading, and deleting files.
Whether you're an old-school C# developer or just starting, this guide offers a clear path to integrate SFTP To Go into your C# projects.
1. Requirements
Before diving into implementing SFTP functionalities in your C# application, some requirements must be met for a smooth and successful integration.
Here's what you need to get started:
.NET development environment:
- Framework: Ensure you have a .NET development environment set up, ideally with the latest version of .NET Core or .NET Framework. This is the platform on which your C# application will be built and run.
IDE: A suitable Integrated Development Environment (IDE) like Visual Studio or Visual Studio Code should be in place. These IDEs offer comprehensive tools and features to aid in your development process, including built-in support for C# and .NET.
SSH.NET library:
- Purpose: SSH.NET is a widely used .NET library that provides functionalities for handling SSH (Secure Shell) and SFTP (Secure File Transfer Protocol) in .NET applications. It is a vital component for implementing SFTP operations in your project.
- Installation: Install SSH.NET using the NuGet Package Manager via the command line interface. The command for installation is:
dotnet add package SSH.NET --version 2020.0.0-beta1
This command adds the SSH.NET library to your project, equipping it with the necessary tools to handle SFTP operations.
Understanding of SFTP and SSH:
- SFTP knowledge: A basic understanding of what SFTP is and how it operates is beneficial. SFTP, unlike standard FTP, ensures that data transfer is encrypted and secure.
- SSH familiarity: Familiarity with SSH (Secure Shell) is also advantageous as SFTP extends the SSH protocol.
Credentials for SFTP server access:
- Credentials: You'll need access to an SFTP server (if you use SFTP To Go, this will be the SFTP To Go server) with the necessary credentials, including the host address, port number, username, and password.
- Storing credentials: Securely store your SFTP To Go credentials in environment variables. This can be done directly in your operating system or via a .env file in your project.
- Loading credentials in C#: Use the DotNetEnv library to load environment variables from a .env file. Retrieve the SFTP To Go URL from the environment variables using Environment.GetEnvironmentVariable("SFTPTOGO_URL").
- Extracting connection details: Parse the SFTP URL to extract the host, port, username, and password. Use the Uri class in C# to split the URL into its components.
using System;
using DotNetEnv;
class Program
{
static void Main()
{
DotNetEnv.Env.Load(); // Load .env file
string sftpUrl = Environment.GetEnvironmentVariable("SFTPTOGO_URL");
var uri = new Uri(sftpUrl);
// Extracting credentials
string host = uri.Host;
int port = uri.Port;
string username = uri.UserInfo.Split(':')[0];
string password = uri.UserInfo.Split(':')[1];
// Credentials can now be used for SFTP operations
}
}
- Key based authentication: In some cases, key-based authentication might be used instead of a password.
Basic C# programming skills:
- C# Language: Basic proficiency in C# programming must follow along with the implementation.
- .NET Concepts: Familiarity with common .NET concepts and constructs, as you'll work with classes, methods, exception handling, and logging.
With these requirements in place, you'll be well-prepared to integrate SFTP functionalities into your .NET applications, enhancing their capabilities to manage file transfers with external servers securely.
2. SftpService class for SFTP operations in C#
The SftpService class is the cornerstone for implementing SFTP operations within your .NET applications. This class encapsulates the functionality needed to interact with an SFTP server, providing a streamlined and secure approach for file operations like uploading, downloading, listing, and deleting files.
Class structure and dependencies:
The SftpService class should be designed to handle various file operations. It relies on the SSH.NET library, which facilitates the interactions with the SFTP server. The class should include:
Dependencies:
- ILogger<SftpService> for logging operations and error handling.
- SftpConfig for storing the SFTP server configuration details like host, port, username, and password.
Constructor:
- The constructor initializes the service with the logger and SFTP configuration.
Methods for file operations:
Each method within SftpService corresponds to a specific SFTP operation:
- ListAllFiles: Lists all files in a specified remote directory.
- UploadFile: Uploads a file from a local path to the remote SFTP server.
- DownloadFile: Downloads a file from the remote SFTP server to a local path.
- DeleteFile: Deletes a file from the remote SFTP server.
Sample implementation:
Here's a simplified version of the SftpService class:
public class SftpService : ISftpService
{
private readonly ILogger<SftpService> _logger;
private readonly SftpConfig _config;
public SftpService(ILogger<SftpService> logger, SftpConfig sftpConfig)
{
_logger = logger;
_config = sftpConfig;
}
public IEnumerable<ISftpFile> ListAllFiles(string remoteDirectory = ".")
{
var client = new SftpClient(_config.Host, _config.Port,
_config.UserName, _config.Password);
client.Connect();
Console.WriteLine("Connected!");
return client.ListDirectory(remoteDirectory);
}
public void UploadFile(string localFilePath, string remoteFilePath)
{
var client = new SftpClient(_config.Host, _config.Port == 0 ? 22 : _config.Port,
_config.UserName, _config.Password);
client.Connect();
var s = File.OpenRead(localFilePath);
client.UploadFile(s, remoteFilePath);
}
public void DownloadFile(string remoteFilePath, string localFilePath)
{
var client = new SftpClient(_config.Host, _config.Port == 0 ? 22 : _config.Port,
_config.UserName, _config.Password);
client.Connect();
var s = File.Create(localFilePath);
client.DownloadFile(remoteFilePath, s);
Console.WriteLine($"Finished downloading file!");
}
public void DeleteFile(string remoteFilePath)
{
var client = new SftpClient(_config.Host, _config.Port == 0 ? 22 : _config.Port,
_config.UserName, _config.Password);
client.Connect();
client.DeleteFile(remoteFilePath);
Console.WriteLine($"File [{remoteFilePath}] deleted.");
}
Integration with .NET applications:
Integrate SftpService into your .NET applications by:
- Instantiating the class: Create an instance of SftpService, passing in the necessary logger and configuration.
- Using the methods: Utilize the methods for various file operations with the SFTP server.
Configuring SFTP in C#:
Configuring SFTP correctly is fundamental in ensuring secure and effective communication between your C# application and an SFTP server (such as the SFTP To Go server). This involves setting up the necessary parameters that allow your application to connect to and interact with the SFTP server. Here's how to go about it:
Understanding SFTP configuration requirements:
SFTP configuration requires specific details about the SFTP server. These details include:
- Host: The address of the SFTP server. If you use SFTP To Go, this will be the SFTP To Go server.
- Port: The port number used for SFTP connections.
- Username: The username for logging into the SFTP server.
- Password: The password associated with the username. Alternatively, key-based authentication can be used.
Creating the SftpConfig class:
The SftpConfig class holds the configuration details. It's a straightforward class with properties corresponding to the necessary SFTP server details:
public class SftpConfig{
public string Host { get; set; }
public int Port { get; set; }
public string UserName { get; set; }
public string Password { get; set; }
Populating configuration details:
You can populate the SftpConfig object in various ways depending on the sensitivity of the information and the application's architecture:
- Hardcoding (not recommended for production): Directly setting values in code.
- Environment variables: Store details in environment variables and read them in your application. This option was outlined in the requirements section of this post.
- Secure vault services: In cloud applications, using services like Azure Key Vault for storing sensitive data.
Example of configuration setup:
Here's an example of setting up the SftpConfig using environment variables:
var config = new SftpConfig{
Host = "your_sftp_server.com",
Port = 22, // Default SFTP port
UserName = "your_username",
Password = "your_password"
}
Utilizing the configuration:
Once you have the SftpConfig object populated, pass it to the SftpService class:
var sftpService = new SftpService(new NullLogger<SftpService>(), config);
3. Performing SFTP operations with SftpService in C#
Once you have configured the SftpService into your C# application, you can begin performing various SFTP operations. These operations are essential for managing files on a remote SFTP server. Here's a comprehensive guide on using SftpService to perform key SFTP operations:
Listing files:
To list files in a directory on the SFTP server:
var files = sftpService.ListAllFiles("/");
foreach (var file in files){
if (file.IsDirectory){
Console.WriteLine($"Directory: [{file.FullName}]");
}
else if (file.IsRegularFile){
Console.WriteLine($"File: [{file.FullName}]");
}
}
Downloading a file:
To download a file from the server to your system:
const string pngFile = @"test.png";
File.Delete(pngFile);
sftpService.DownloadFile(@"/folder/imap-console-client.png", pngFile);
if (File.Exists(pngFile)){
Console.WriteLine($"file {pngFile} downloaded");
}
Uploading a file:
To upload a file from your system to the SFTP server:
var testFile = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "test.txt");
sftpService.UploadFile(testFile, @"/folder/test.txt");
Deleting a file:
Simply use the below statement to delete a file from the SFTP server:
sftpService.DeleteFile(@"/folder/test.txt");
Exception handling and logging:
Each operation is wrapped in a try-catch block to handle any exceptions during the SFTP operations. Proper logging of these exceptions is critical for diagnosing issues in production.
Connection management:
The SftpService class is designed to handle the connection lifecycle within each method, ensuring that connections are opened and closed as needed. This avoids leaving connections open unnecessarily and handles any connection issues that may arise during an operation.
To close the connection, use the Disconnect method that will be called using the client object formally created in the SftpService class while calling the operations methods:
client.Disconnect();
4. The whole thing
You now have a comprehensive understanding of how to set up and use the SftpService for secure file operations with SFTP in C#. You might want to run the entire program from start to finish to put all these pieces together and see the full implementation in action.
The complete code, including the SftpService class, SftpConfig settings, and examples of various SFTP operations (like listing files, uploading, downloading, and deleting), is available for your reference and use.
Accessing the full code:
To access and run the complete program, visit the SFTP To Go Examples GitHub repository. The repository contains all the code discussed in this article, neatly organized and ready to be executed. Here's how you can access it:
- Visit the GitHub repository: Getting Started with SFTP using C#(.NET)
- Review and download: You can review the code directly on GitHub. To run it on your machine, download the entire project or clone the repository using Git:
git clone https://github.com/crazyantlabs/sftptogo-examples.git
- Navigate to the dotnet Subdirectory: Once the repository is cloned, you can navigate to the dotnet subdirectory within the cloned repository:
cd sftptogo-examples/dotnet
- Open and run: Open the downloaded project in your preferred .NET IDE and run it to see SftpService in action.
Conclusion: integrating SFTP into your C# workflow
SFTP To Go provides a secure and efficient way to manage your file transfers, and integrating it with C# opens up a wide range of possibilities for your applications.
Whether it's automating backups, sharing data between systems, or securely handling sensitive information, these skills are an essential part of modern software development.
Remember, the examples provided are just starting points. Depending on your specific needs, you might want to explore more advanced features of the SSH.NET library, error handling, or even automating these processes.
The journey through the world of secure file transfers in C# is just beginning, and there's much more to explore and implement.
Looking to connect SFTP in Python instead? Go Here.
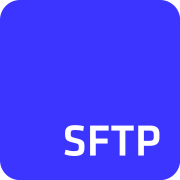
Frequently Asked Questions
Do I need special permissions or setup to use SFTP To Go with my C# application?
To use SFTP To Go, you need access to an SFTP server with valid credentials (username, password, and host details). No special permissions are usually required beyond what's needed to set up a regular SFTP connection.
Is it difficult to integrate SFTP To Go into an existing C# application?
Integrating SFTP To Go into an existing C# application is straightforward, especially with libraries like SSH.NET. The SftpService class outlined in this guide provides a clear template for implementing and utilizing SFTP functionalities.
How does error handling work in SFTP operations in C#?
Error handling in SFTP operations involves catching exceptions during file operations and logging them for review. This allows for informed troubleshooting and ensures the application can gracefully handle unexpected issues.
Are there any limitations I should be aware of when using SFTP in C#?
The primary limitations to be aware of when using SFTP in C# are related to network speed and server configurations, such as bandwidth limits or file size restrictions set on the server. The performance may also depend on the efficiency of the implemented SFTP logic in your application.
How do I handle connection failures or timeouts in SFTP operations?
Connection failures or timeouts should be handled through exception handling and retry logic. Implementing retries with exponential backoff strategies can effectively manage network issues.